Authorize.net has a user base of over 200k merchants making it the largest payment gateway service provider. Most e-commerce solutions already integrate with Authorize.net, including our favorite e-commerce store Satchmo, developed in django, that we have covered earlier.
However, many shopping portals still require custom development. The robust REST API Authorize.net offers (AIM and SIM) allows for integration with e-commerce merchants’ websites.
The AIM API allows for the check out of the customer within the merchant’s site, it requires SSL certificate for the merchants site and data is to be transferred in an 128-bit encrypted format.
The SIM API on the other hand allows for a hosted check out from Authorize.net’s site. The appearance, look and feel, CSS, logos, header and footer of their site can be customised, so that users experience a similar interface.
Thus a merchant can perform, based on his business need, one of the following:
- Using the SIM API, perform the checkout and display the receipt on Authorize.net
- Using the SIM API perform the checkout on Authorize.net and display the receipt on merchant's site, by using relay response
- Using the SIM API obtain Authorization confirmation on Authorize.net and perform the checkout and display the recipt on the merchant's site
- Using AIM API, perform the entire checkout process on merchant's site
The third option above is interesting. The Authorize.net’s SIM provides the flexibility to checkout on merchant’s site for transactions, even without SSL, for the cost of a round trip of http handshakes, just like the Paypal’s Express Checkout API.
Typically, users return back to the merchant site and where the receipt is displayed (case 2) or confirm at the merchant site (case 3). Lets examine the workflow for such a scenario.
- Find the total amount payable by user, all inclusive. (incl taxes, shipping)
- Generate the fingerprint of transaction, based on merchant login-id, invoice-number, time-stamp and amount using the MD5 hashing library.
- Pre-populate all the hidden input form fields for the transaction on the template. (covered in detail, below)
- Send the user to Authorize.net when they submit the form, with pre-populated values
- After the authentication, the response is posted to merchant site
- Verify for success in the response.
- If you opted for payment on the authorize.net site itself, display the receipt.
- If you have opted for check out at your site, Confirm payment from the user and submit a new request of `x_type = 'PRIOR_AUTH_CAPTURE'`, also include the `x_trans_id` obtained in the response. (preferably as an AJAX request)
- If error occurs or if customer cancels, submit a void request.
The integration essentially involves, sending a list of hidden fields in a form to the specified url:
- Include the following Required fields in the form, set to appropriate values.
x_fp_hash
The fingerprint
x_fp_timestamp
UTC time in seconds since epoch
x_fp_sequence
Invoice number, or a random number
x_login
Login ID of merchant, provided by Authorize.net
x_show_form
TRUE, to show form
x_amount
Total Amount of the transaction
- Set the response type, `x_relay_response` to TRUE, and `url_response` to the url that to which POST has to be posted- We prefer to use the same url which is submitting the request.
- Set the `x_type` to `Auth_only` for a checkout at your site, or `Auth_capture` for a checkout at Authorize.net
- Include Additional fields, where appropriate. Entire list of fields
- Set the form submit to the Authorize.net specified url. Submissions go to: https://secure.authorize.net/gateway/transact.dll
The following view code should indicate how you should go about doing it:
import hmac,time,urllib,urllib2
def payment1(req):
if (req.method == 'GET'):
#Use these values in the template to pre-populate a form that submits
#these hidded fields to url http://secure.authorize.net/gateway/transact.dll
payload = {
'x_login' : 'login-id',
'x_amount' : '100.00',
'x_show_form' : 'PAYMENT_FORM',
'x_type' : 'AUTH_CAPTURE',
'x_method' : 'CC',
'x_fp_sequence' : '101',
'x_version' : '3.1',
'x_relay_response' : 'TRUE',
'x_fp_timestamp' : str(int(time.time())),
#The same Url as the current one, whatever it is.
'x_relay_url' : reverse("payment_url")
}
msg = '^'.join([params['x_login'],
params['x_fp_sequence'],
params['x_fp_timestamp'],
params['x_amount']
])+'^'
fingerprint = hmac.new('9LyEU8t87h9Hj49Y',msg).hexdigest()
payload['x_fp_hash'] = fingerprint
return render_to_response('template1.html', payload, RequestContext(request))
else if (req.method == 'POST'):
#Handle the response, Verify POST dictionary
if(req.post[x_response_code] == 1) :
#Success
#Display the receipt or
#Confirm from the user
pass
Looking to develop an e-commerce website? We offer services. Get in touch.
Thank you for reading the Agiliq blog. This article was written by lakshman on Mar 31, 2009 in ecommerce .
You can subscribe ⚛ to our blog.
We love building amazing apps for web and mobile for our clients. If you are looking for development help, contact us today ✉.
Would you like to download 10+ free Django and Python books? Get them here
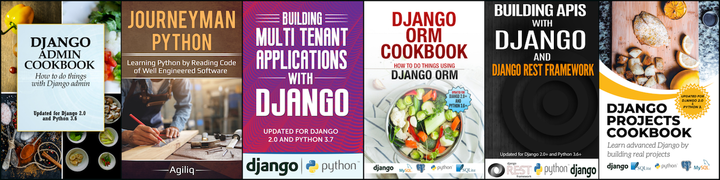